For the past few years, if you needed ServiceNow to send a SOAP request to a third-party web service that was behind a firewall, you needed to establish a VPN connection between your ServiceNow instance and the third-party Web service.
As of a recent release in 2011 all that has changed! Built by popular demand, ServiceNow has the ability to send SOAP messages through the ServiceNow mid server.
For the most part, the consuming of a SOAP web service through the MID server is the same as connecting directly. However, there are a few differences which I would like to highlight.
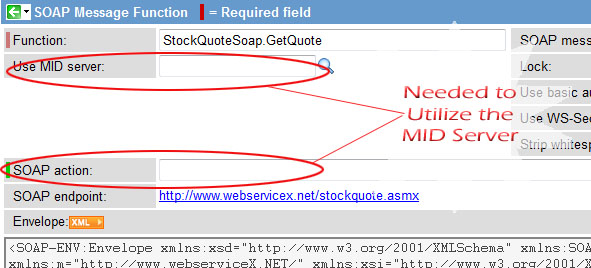
When creating your SOAP message, make sure you select a MID Server and that the SOAP Action field is filled out properly.
First of all, it is pretty obvious, but you need to select a MID Server for the SOAP Message function. This is set at the Function level, not at the message level.
Second, usually the SOAP Action field is already filled out when you populate the function based off of a WSDL. However, in some cases, the SOAP Action field is blank. If this is the case, you need to fill this out appropriately. It should be the URL for that SOAP function. It can be found in the WSDL.
Probably the biggest difference between consuming a SOAP interface directly and going through the MID Server is that since we are going through the MID Server all of the SOAP requests will be asynchronous. This means you won’t be able to get the response in the same way as if you were performing synchronous SOAP calls.
Let’s try it out.
First, I am going to use the sample “Weather Forcast” SOAP Message record that is included by default in your instance. I will use the “GetWeatherByZipCode” function.
The following is my SOAP Message Function Record:
As you can see, my MID Server is named “RadRoadTrips.com” after one of my favorite family travel websites. Also, we need to change the Zipcode element value string to be a variable…I named my variable: “${zipcode}”.
Now, that we have this set up, we can go directly into scripting. The scripting is going to be the same as you are familiar with already for the SOAP consumer in ServiceNow. As I mentioned before, the biggest change in the script is how we fetch the response.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | var s = new SOAPMessage('WeatherForecast', 'WeatherForecastSoap.GetWeatherByZipCode'); s.setParameter('zipcode', '83274'); s.post(); var k = 1; var r = s.getResponse(); while(r == null) { gs.log("waiting ... " + k + " seconds"); r = s.getResponse(1000); k++; if (k > 330) { break; // service did not respond after 30 tries } } doc = new XMLDocument(r); gs.log(doc.getNodeText("//WeatherData[1]/MaxTemperatureF")); |
This code uses a new function on the Soap object called “getResponse”. This function takes in an optional parameter that waits X number of milliseconds before querying the ECC Queue for a response to the SOAP request.
In this code snippet, I loop through, checking every 1 second for a maximum of 330 seconds to see if there is a response. Once I get a response, I grab today’s max temperature using the following XPath statement:
“//WeatherData[1]/MaxTemperatureF”.
If you go to the ECC Queue, you can see your SOAP transactions, both request and response.
One other option that I would like to cover is the capability of setting the MID Server in your script rather than just in the record. This is handy if you have multiple mid servers or you have some functions that require a mid server and others that don’t.
To set your MID Server, you just need to use the following code:
1 2 3 4 | var s = new SOAPMessage('WeatherForecast', 'WeatherForecastSoap.GetWeatherByZipCode'); s.setParameter('zipcode', '83274'); s.setMIDServer("RadRoadTrips.com"); s.post(); |
Hopefully you’re still monitoring these comments, even though this is a very old post.
Basically, I’m wondering how this works when the WSDL and web service you need to consume sits behind a firewall. I can copy and paste the WSDL into a SOAP message function record, but get an error when I try to create SOAP message functions because – I believe it’s trying to look for a schemaLocation and/or endpoint that’s behind the firewall (i.e., accessible via the MID Server).
My other question is regarding the MID Server option on a SOAP message function record. Does there have to be a configured MID Server for that field to appear? Right now, I don’t have that option in my instance even though the MID Server plugin is enabled.
Hi John,
As suggested, when I execute my business rule script using setMIDServer parameter, my message does not reach MID Server and I come up with below response in ecc queue:
===========================
results error=”java.net.SocketTimeoutException: connect timed out when posting to given end point” probe_time=”10000″
===========================
Even before executing my script, I was just wondering how system will login to MID Server by using only setMIDServer parameter and no username and password.
Not sure, if response received in ecc queue is because of this only.
Can you please suggest something?
Brgds, AM